Google developed the Dart programming language and released it in 2011 and 2013. Dart is a language designed to build applications for mobile, web, and server environments. It is an object-oriented programming (OOP) language with a class-based structure and a syntax similar to C language. If you are familiar with languages such as C#, C++, Swift, Kotlin, or Java/JavaScript, you will find it easy to start developing in Dart.
Hello World
Let’s start with a simple code example. The code below prints “Hello, World!”. You will notice that there is a main
function, which is the entry point where execution begins. This function has a void
return type, meaning it does not return a value. Inside the main
function, the print()
function is used to display text on the console.
void main() {
print('Hello, World!');
}
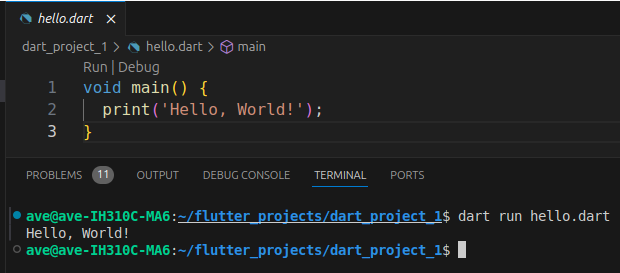
COMMENT
In any application, comments enhance code readability. They describe the logic and dependencies of the application. How do we use comments in Dart?
- Single-line comments begin with //, and the Dart compiler ignores everything to the end of the line.
- Multiline comments begin with /* and end with */. The Dart compiler ignores everything between the slashes.
- Documentation comments begin with ///, and the Dart compiler ignores everything to the end of the line unless enclosed in brackets.
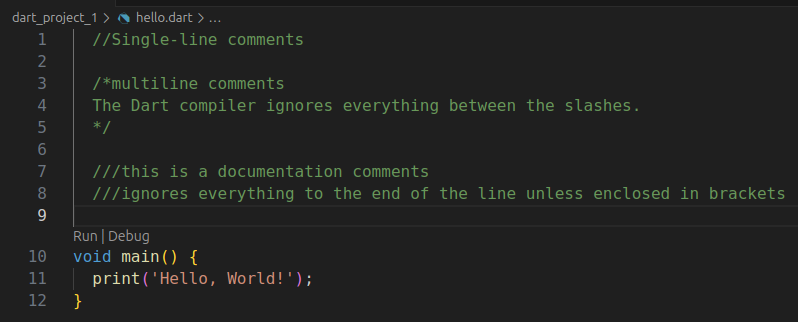
DECLARING VARIABLES
In Dart, all variables are publicly accessible by default, meaning they can be accessed by everyone. If you want to make a variable private, you can start its name with an underscore (_). By doing this, you’re indicating that the variable cannot be accessed from outside the class or function where it is declared; in other words, it can only be used within that specific class or function.
You can also declare a variable with an implicit type. For example, if you declare a variable named myVariable and assign “Sarah” without specifying its type, it will be inferred as a String type. You can change the value of myVariable, but it must always be a String, which is a sequence of characters.
var myName = "Sarah";
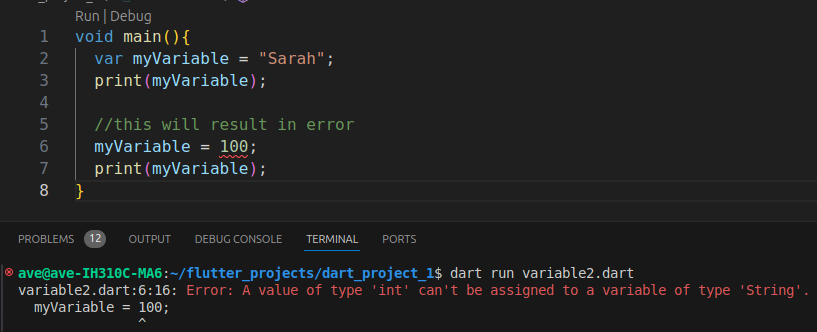
If an object isn’t restricted to a single type, specify the Object
type (or dynamic
if necessary).
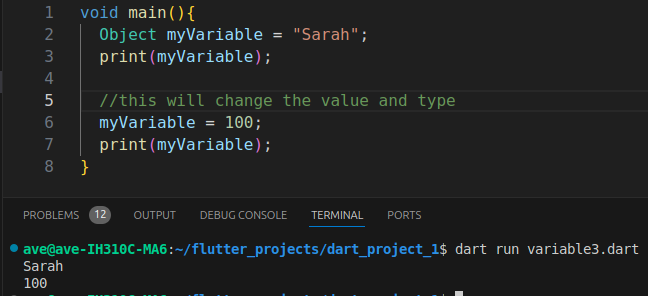
Strings
A string is a sequence of text characters. It can be a single line of characters, which can be enclosed in either single quotes (like ‘Jane Doe’) or double quotes (like “Jane Doe”). For multiline strings, use triple quotes, for example: ”’John is an artist, a smart student, and also somewhat melancholy.”’ You can concatenate (combine) two or more strings by using the plus (+) operator or by placing adjacent single or double quotes together.
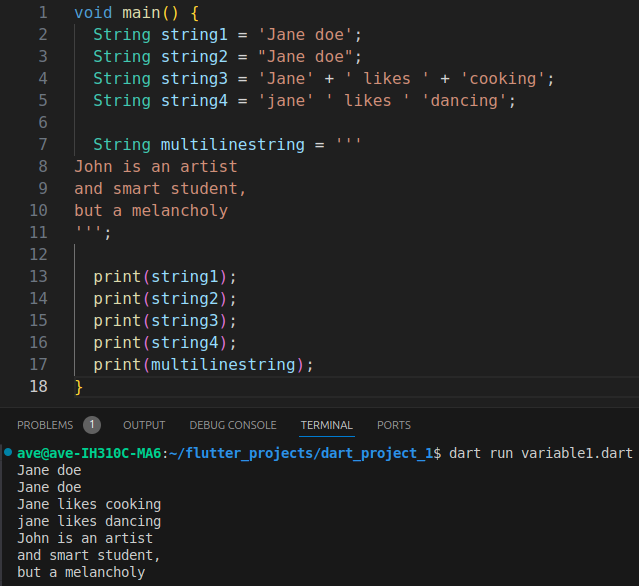
Numbers
Dart represents numbers in few ways:
- Int: 64-bit signed non-fractional integer values such as -2 to 2 -1.
- Double: Dart represents fractional numeric values with a 64-bit double-precision floating-point number.
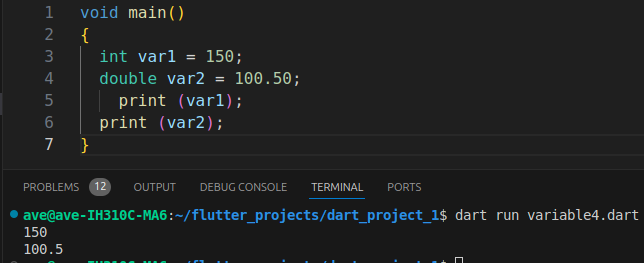
Booleans
Dart provides the bool
type, which can only have two values: true and false. In the example below, we have two boolean variables: isRaining
, which is set to true, and bringUmbrella
, which is set to false. The logic is as follows:
- If the variable
isRaining
is true, the program should print “It’s raining.” - If the variable
bringUmbrella
is true, the program should print “Use umbrella.”
Therefore, the output will only be “It’s raining,” as that is the only variable with a true value.
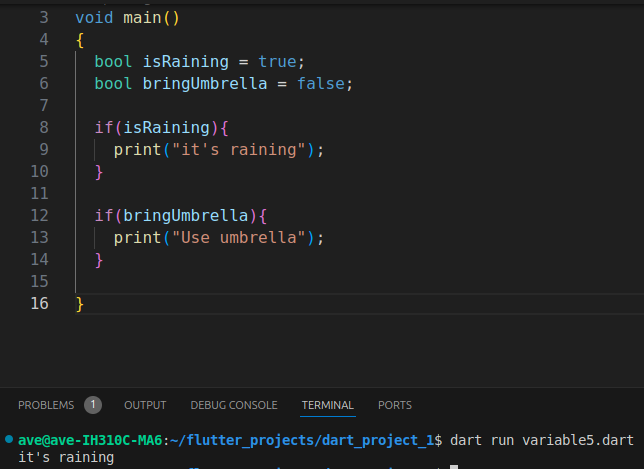
Null safety
Null safety helps prevent errors that arise from unintentionally accessing variables that are set to null. This type of error is known as a null dereference error, which occurs when you try to access a property or call a method on an expression that evaluates to null. Exceptions to this rule arise when null is a valid input for the property or method, such as with toString() or hashCode. With null safety, the Dart compiler can detect these potential errors during compile time.
Null safety introduces three key changes:
Type Declaration and Nullability: When you specify a type for a variable, parameter, or another relevant component, you can control whether that type accepts null values. To indicate that a type is nullable, you add a ?
to the end of the type declaration.
In the code example below, the variable name1 can accept null values without causing an error. In contrast, the variable name2 cannot accept null values and will raise an error if it does.

Variable Initialization: You must initialize all variables before using them. Nullable variables default to null
, so they are initialized by default. However, Dart does not assign initial values to non-nullable types, requiring you to explicitly set an initial value. You cannot access an uninitialized variable, which helps prevent errors when trying to access properties or call methods on types that could potentially be null
.
Accessing Properties and Methods: You cannot access properties or call methods on expressions with a nullable type. The same restriction applies to properties or methods that null
supports, such as hashCode
or toString()
.
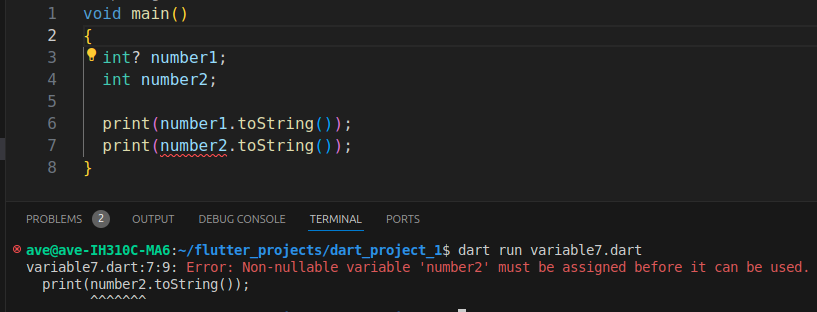
Default value
Uninitialized variables that have a nullable type have an initial value of null
. Even variables with numeric types are initially null, because numbers and everything else in Dart are objects.
Final and const
If you want a variable’s value to remain constant, start the declaration with either final or const. Use final when the value is assigned at runtime and can be changed by the user. Use const when the value is known at compile time and will not change during runtime.
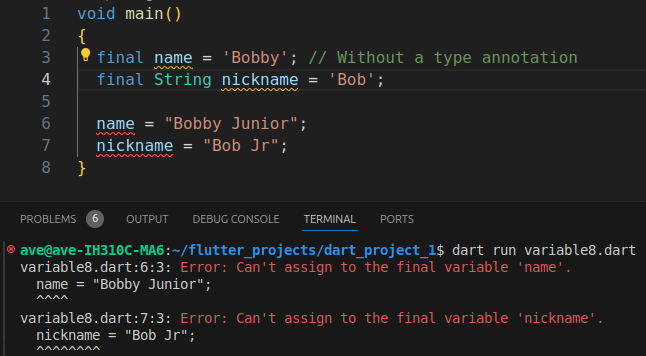
You can change the value of a constant variabel like this code below.
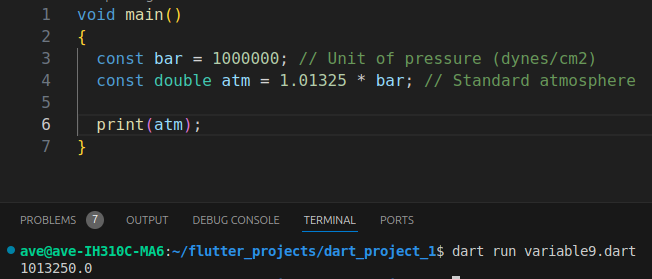
But this code below will raise an error.
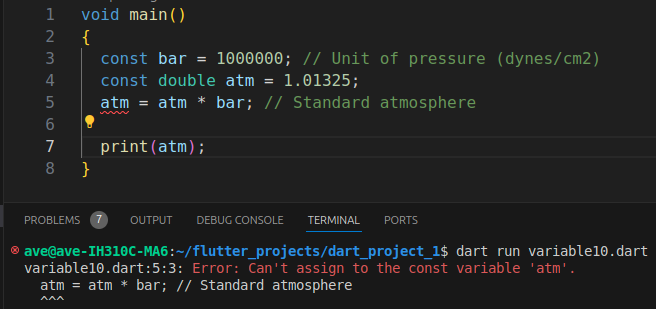