Retrieving Data from websites with HTTP GET in Flutter
GET requests are used to extract data from the backend for use in the application. To make a GET request from a website with Flutter, here are the steps.
Get the dart HTTP package
Open a new terminal then type
flutter pub add http.

Add permission to access the internet in AndroidManifest.xml
We have to add permission to access the internet in AndroidManifest.xml. This file is in the android > app > main > AndroidManifest.xml folder.
Then add the code below below the tag but before the tag
<uses-permission android:name="android.permission.INTERNET" />
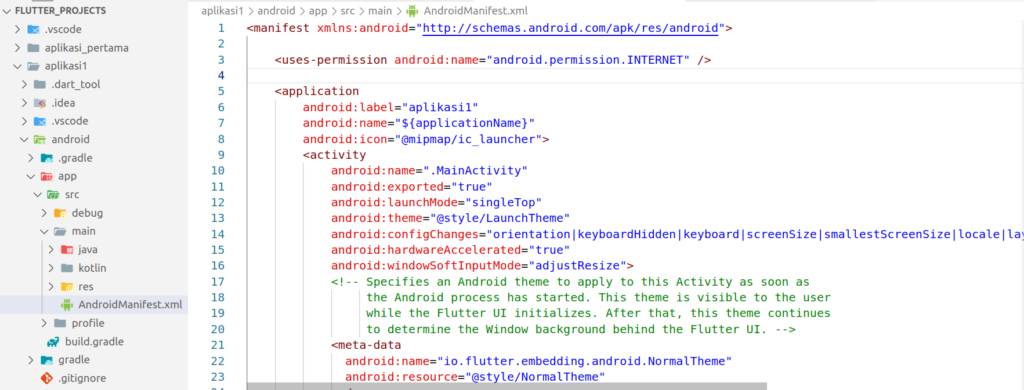
The following is the data that we will retrieve from the server. There is a website that provides data to be get for free, which is https://jsonplaceholder.typicode.com/.
We will retrieve a post-like data so change the URL to https://jsonplaceholder.typicode.com/posts .
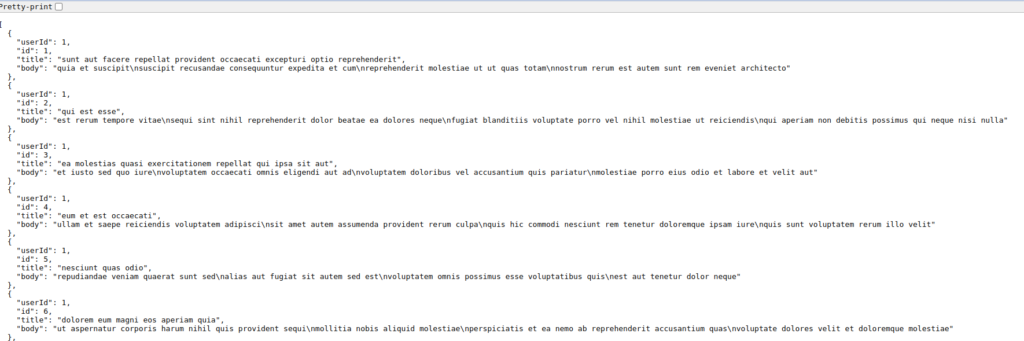
We will start with a simple code to get data first.
import 'package:http/http.dart' as http;
import 'dart:convert';
import 'package:flutter/foundation.dart';
void main() async {
List posts = [];
try {
const apiUrl = 'https://jsonplaceholder.typicode.com/posts';
final http.Response response = await http.get(Uri.parse(apiUrl));
posts = json.decode(response.body);
posts.forEach((element) => print("tile:" + element['title']));
} catch (err) {
if (kDebugMode) {
print(err);
}
}
}
We can see here that the post data is taken from the website jsonplaceholder.typicode.com. Data retrieved with HTTP is in the form of text. This text data needs to be converted to JSON form to make it easier to read and store in the posts variable. Then, using a loop, we read the post data and print only the title contents. Because only the title is printed, we must retrieve it by specifying the column name like element[‘title’]. The results will be printed on the terminal as shown below.
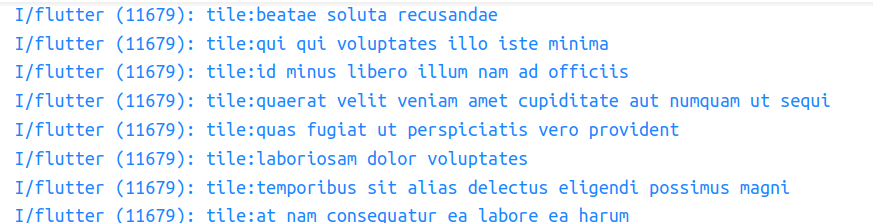
Making User Interface
In the following we will display the title and body of the post data in a list.
import 'package:flutter/material.dart';
import 'package:http/http.dart' as http;
import 'dart:convert';
import 'package:flutter/foundation.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
debugShowCheckedModeBanner: false,
home: PartHome(),
);
}
}
class PartHome extends StatefulWidget {
const PartHome({super.key});
@override
State<PartHome> createState() => PartHomeState();
}
class PartHomeState extends State<PartHome> {
Future<List> ambilData() async {
List posts = [];
try {
const apiUrl = 'https://jsonplaceholder.typicode.com/posts/';
final http.Response response = await http.get(Uri.parse(apiUrl));
posts = json.decode(response.body);
} catch (err) {
if (kDebugMode) {
print(err);
}
}
return posts;
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text("GET DATA FROM WEBSITE"),
),
body: Column(children: [
Expanded(
child: FutureBuilder(
future: ambilData(),
builder: (BuildContext ctx, AsyncSnapshot<List> snapshot) =>
snapshot.hasData
? ListView.builder(
// render the list
itemCount: snapshot.data!.length,
scrollDirection: Axis.vertical,
itemBuilder: (BuildContext context, index) => Column(
children: [
Text(snapshot.data![index]['title']),
Text(snapshot.data![index]['body'])
],
))
: const Center(
// render the loading indicator
child: Text("NOT FOUND"))),
),
]),
);
}
}
Output:
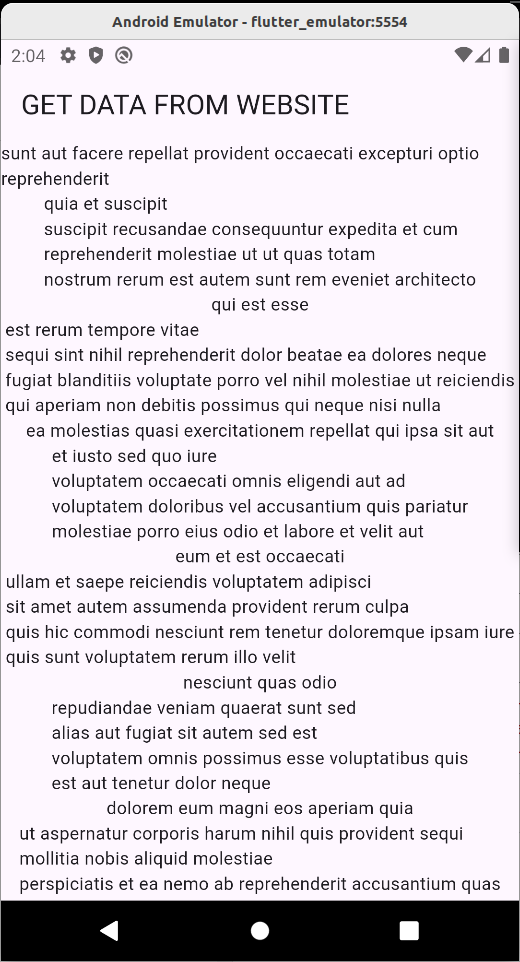
You can see the title and body displayed in a vertical list. But the appearance is still a little untidy, let’s add cards and padding to tidy up the appearance.
import 'package:flutter/material.dart';
import 'package:http/http.dart' as http;
import 'dart:convert';
import 'package:flutter/foundation.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
debugShowCheckedModeBanner: false,
home: PartHome(),
);
}
}
class PartHome extends StatefulWidget {
const PartHome({super.key});
@override
State<PartHome> createState() => PartHomeState();
}
class PartHomeState extends State<PartHome> {
Future<List> ambilData() async {
List posts = [];
try {
const apiUrl = 'https://jsonplaceholder.typicode.com/posts/';
final http.Response response = await http.get(Uri.parse(apiUrl));
posts = json.decode(response.body);
} catch (err) {
if (kDebugMode) {
print(err);
}
}
return posts;
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text("GET DATA FROM WEBSITE"),
),
body: Column(children: [
Expanded(
child: FutureBuilder(
future: ambilData(),
builder: (BuildContext ctx, AsyncSnapshot<List> snapshot) =>
snapshot.hasData
? ListView.builder(
itemCount: snapshot.data!.length,
scrollDirection: Axis.vertical,
itemBuilder: (BuildContext context, index) => Column(
children: [
Padding(
padding: const EdgeInsets.all(7),
child: Card(
elevation: 10,
child: Padding(
padding: const EdgeInsets.all(7),
child: Column(
children: [
Align(
alignment: Alignment.topLeft,
child: Text(
snapshot.data![index]
['title'],
style: const TextStyle(
fontSize: 20,
fontWeight:
FontWeight.w700,
)),
),
Align(
alignment: Alignment.topLeft,
child: Text(
snapshot.data![index]
['body'],
style: const TextStyle(
fontSize: 14,
fontWeight:
FontWeight.w300,
)),
)
],
),
)))
],
))
: const Center(child: Text("NOT FOUND"))),
),
]),
);
}
}
Output:
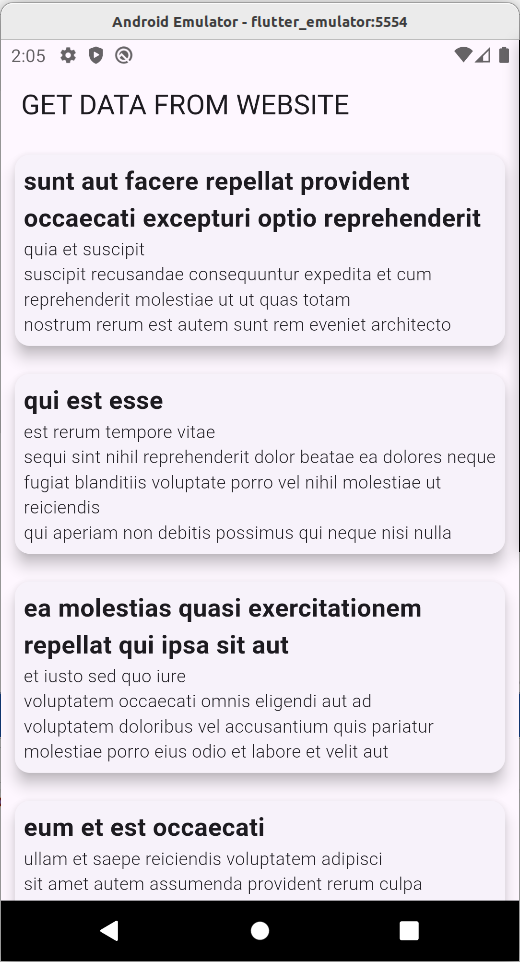